Juniper's PyEZ
Commit, Confirm, Rollback
In the last article, we loaded configuration changes from a file using PyEZ. Now let's look at some other aspects of PyEZ and configuration file management.
First, let's lock the candidate config so that no one else can make changes:
>>> from jnpr.junos import Device
>>> from jnpr.junos.utils.config import Config
>>> from jnpr.junos.exception import LockError
>>>
>>> from pprint import pprint
>>>
>>> a_device = Device(host='10.10.10.10', user='pyclass', password='password')
>>> a_device.open()
Device(10.10.10.10)
>>>
>>> cfg = Config(a_device)
>>> cfg.lock()
True
Now, let's verify this lock in the SRX terminal window:
pyclass@pynet-jnpr-srx1> configure
Entering configuration mode
Users currently editing the configuration:
pyclass (pid 99550) on since 2015-02-18 23:48:44 PST
exclusive
[edit]
pyclass@pynet-jnpr-srx1# set system host-name pytest
error: configuration database locked by:
pyclass terminal (pid 99550) on since 2015-02-18 23:48:44 PST, idle 00:01:06
exclusive
[edit]
pyclass@pynet-jnpr-srx1#
As we would expect, however, we can still make changes using PyEZ:
>>> cfg.load("set system host-name pytest2", format="set", merge=True)
<Element load-configuration-results at 0x285ab00>
Now that locking is working, let's use PyEZ to rollback the configuration. The Juniper CLI shows that one change is pending:
pyclass@pynet-jnpr-srx1# show | compare
[edit system]
- host-name pynet-jnpr-srx1;
+ host-name pytest2;
[edit]
pyclass@pynet-jnpr-srx1#
With PyEZ we can roll this back as follows:
>>> cfg.rollback(0)
True
And the SRX CLI now shows:
pyclass@pynet-jnpr-srx1# show | compare
[edit]
pyclass@pynet-jnpr-srx1#
How about committing a change? First, let's re-execute the hostname change from earlier and then commit it.
>>> cfg.load("set system host-name pytest2", format="set", merge=True)
<Element load-configuration-results at 0x285d128>
>>> print cfg.diff()
[edit system]
- host-name pynet-jnpr-srx1;
+ host-name pytest2;
>>> cfg.commit(comment="Testing commit using PyEZ")
True
The SRX commit history now shows:
pyclass@pytest2> show system commit
0 2015-02-18 23:58:28 PST by pyclass via netconf
Testing commit using PyEZ
1 2015-02-18 23:48:27 PST by pyclass via cli
2 2015-02-18 16:59:00 PST by root via cli
3 2015-02-18 16:58:11 PST by root via cli
4 2015-02-17 05:44:58 PST by root via cli
5 2015-02-17 05:43:46 PST by root via cli
Now that commit is working, let's try a commit-confirm (using a two minute confirm time):
>>> cfg.load("set system host-name pynet-jnpr-srx1", format="set", merge=True)
<Element load-configuration-results at 0x285dcf8>
>>> print cfg.diff()
[edit system]
- host-name pytest2;
+ host-name pynet-jnpr-srx1;
>>> cfg.commit(comment="Testing commit-confirm using PyEZ", confirm=2)
True
Back on the SRX, we can see that the commit will be rolled back in two minutes:
pyclass@pytest2# show | compare
# commit confirmed will be rolled back in 2 minutes
[edit]
pyclass@pynet-jnpr-srx1#
Now we can do our second commit and verify it in the commit history.
>>> cfg.commit()
True
pyclass@pynet-jnpr-srx1> show system commit
0 2015-02-19 00:04:13 PST by pyclass via netconf
1 2015-02-19 00:03:09 PST by pyclass via netconf commit confirmed, rollback in 2mins
Testing commit-confirm using PyEZ
2 2015-02-18 23:58:28 PST by pyclass via netconf
Testing commit using PyEZ
3 2015-02-18 23:48:27 PST by pyclass via cli
4 2015-02-18 16:59:00 PST by root via cli
5 2015-02-18 16:58:11 PST by root via cli
pyclass@pynet-jnpr-srx1>
Now that we are all done, we need to release our lock on the candidate config:
>>> cfg.unlock()
True
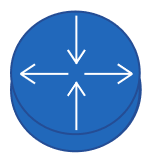
Kirk Byers